Conditional Rendering of Components
Learn how to conditionally render a component in this guide.
Creating a Component
To start, ensure you have your component design ready in Figma. This is the design we'll be using in this guide.
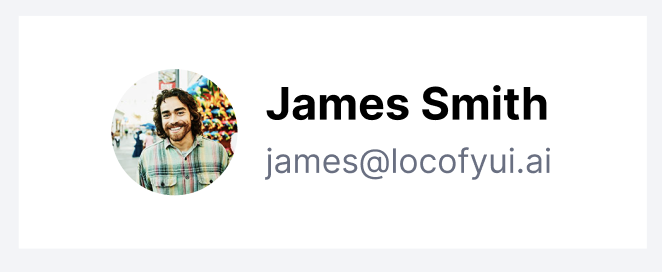
To export the design as a component, follow these steps:
- Select your component.
- Run the Locofy.ai plugin.
- Click "Sync" in Step 5: Sync to Locofy Builder (opens in a new tab).
- Choose "Single Component."
- Start the sync process.
- View the code in the builder.
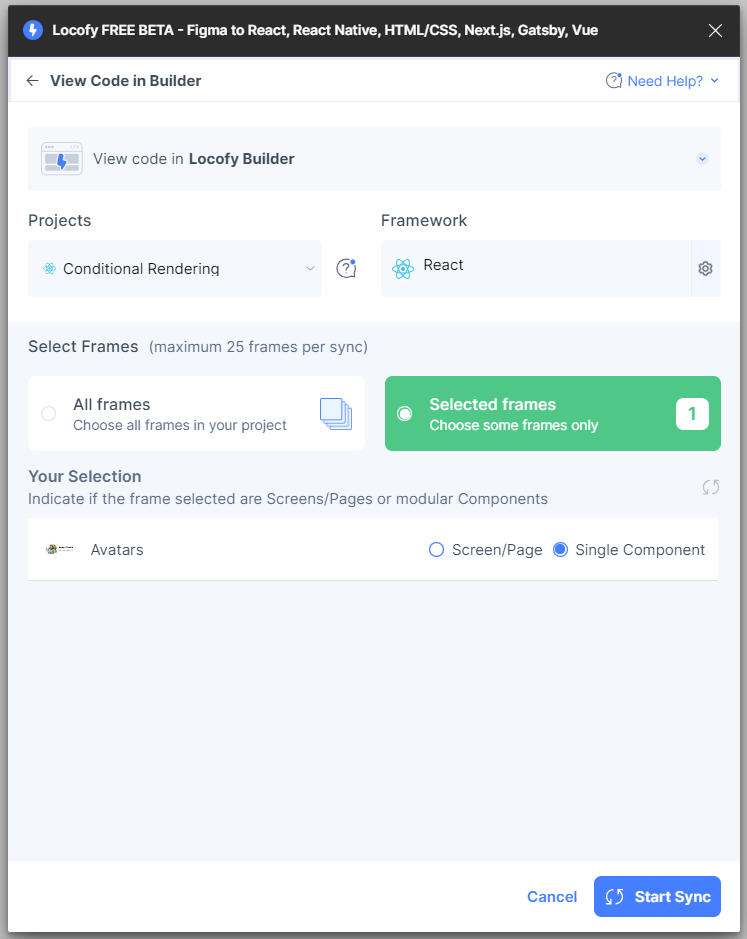
Adding Conditional Rendering to the Component in Builder
In the Locofy.ai builder, your design will be recognized as a component because of how it was exported.
To add conditional rendering, follow the steps below:
-
Click on the "Add Props" button in Step 6: Make Components (opens in a new tab).
-
Select the "badge" prop in our case. You will be presented with different types of props; choose "Conditional Rendering."
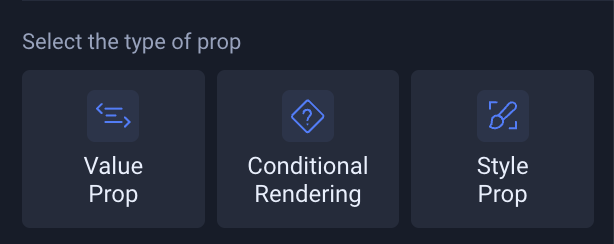
- Once selected, you can add conditions and a default value, then hit the save button.
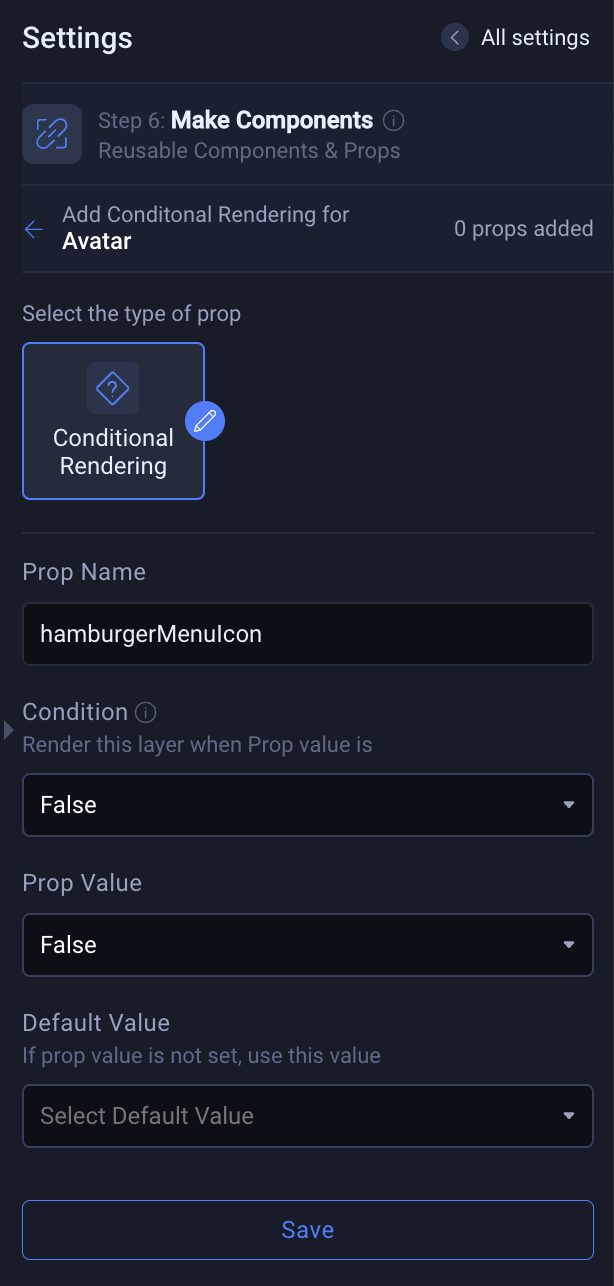
Downloading Our Code
To download or sync the code with your existing app, click "Sync/Export/Deploy" i.e Step 8: Sync/Export/Deploy (opens in a new tab).
Clicking it will reveal available options.
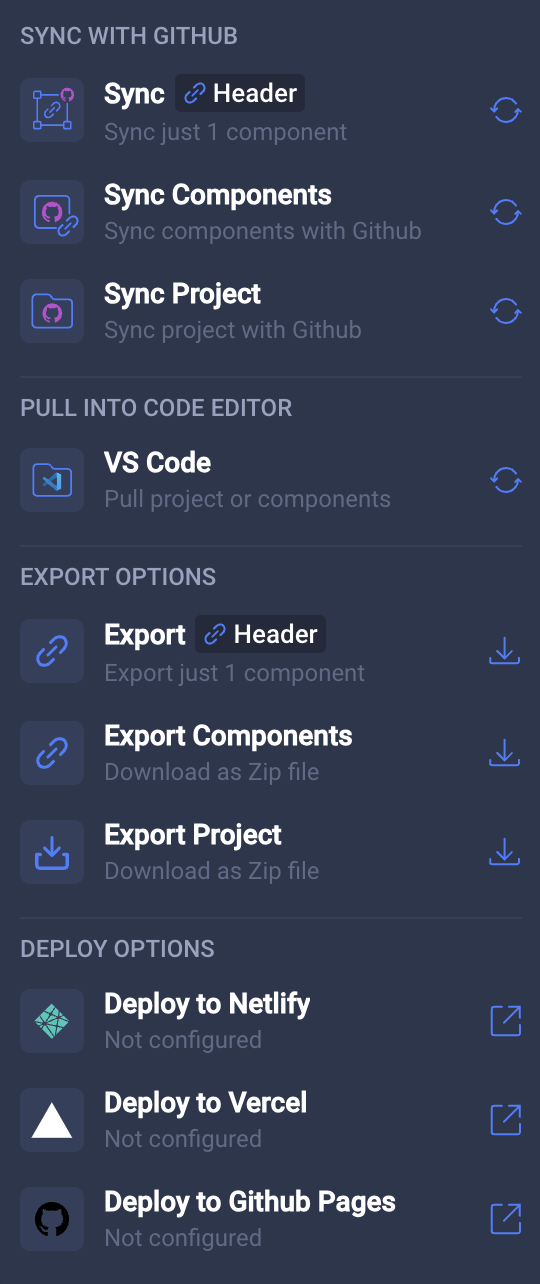
Choose the first export option that just exports the selected component and save it to your local machine. Below is the final code for our component:
import "./Avatar.css";
const Avatar = ({ showBadgeIcon = false }) => {
return (
<div className="avatar">
<div className="imagecontainer">
<img className="image-icon" alt="" src="/image@2x.png" />
{showBadgeIcon && (
<img className="badge-icon" alt="" src="/badge.svg" />
)}
</div>
<div className="avatar-details">
<b className="name">James Smith</b>
<div className="email">james@locofyui.ai</div>
</div>
</div>
);
};
export default Avatar;
The badge will only be rendered when showBadgeIcon
is true
. If the prop is not set, the badge will not be shown.
Conditionally Rendering the Component
Now, let's use our component in an existing React project. We will toggle the badge by checking if a randomly generated number is even or not.
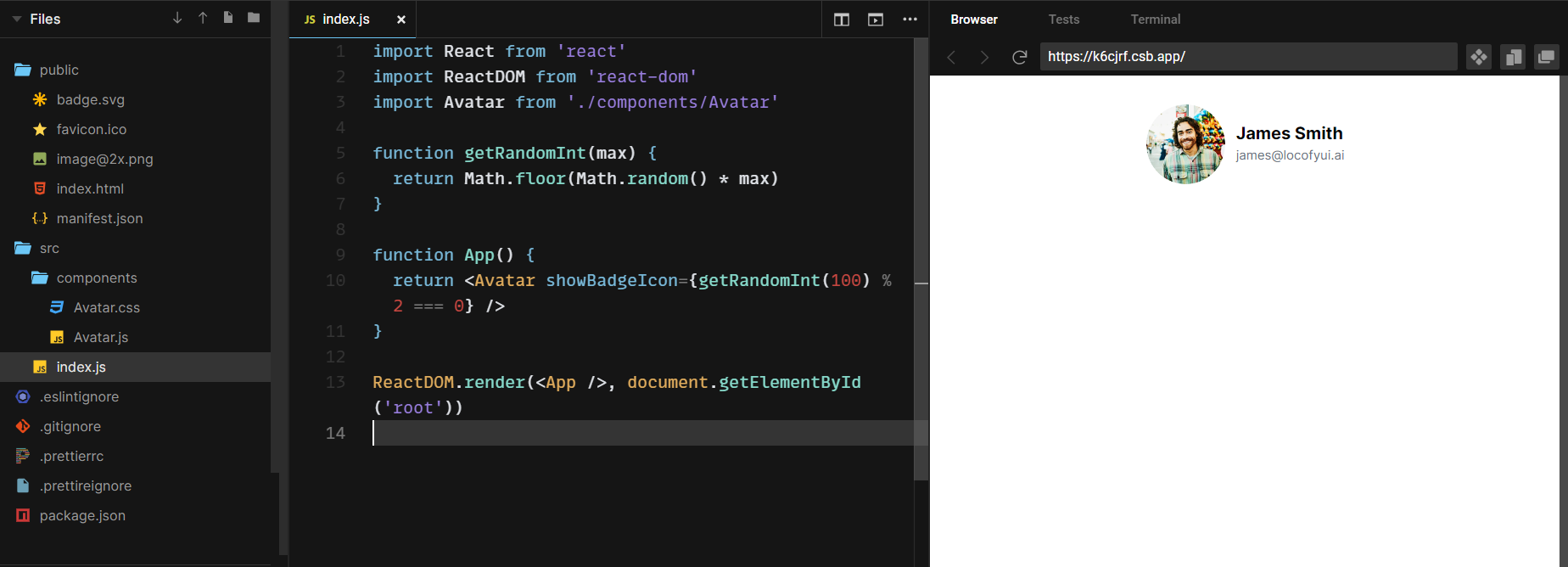
Whenever you refresh the page and the randomly generated number is even, the badge will be displayed.